Activity's Life Cycle
The life cycle of an activity is managed via the following call-back methods, defined in the android.app.Activity
base class:
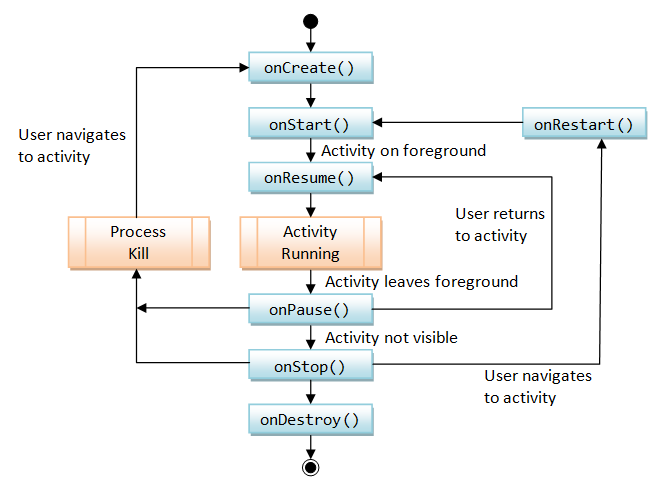
onCreate()
,onDestroy()
: Called back when theActivity
is created/destroyed.onStart()
,onStop()
,onRestart()
: Called back when theActivity
is starting, stopping and re-starting.onPause()
,onResume()
: Called when theActivity
is leaving the foreground and back to the foreground, can be used to release resources or initialize states.
Refer to Android API on Activity
(http://developer.android.com/reference/android/app/Activity.html) for more details.
Example 8: Activity's Life Cycle
To illustrate the activity's life cycle, create a new Android project with application name of "Life Cycle
", and package name "com.example.lifecycle
". Create a activity called "MainActivity
" with layout "activity_main
".
Modify the "MainActivity.java
" as follows:
package ......; import ......; import android.util.Log; public class MainActivity extends ActionBarActivity { private static final String TAG = "MainActivity: "; @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); Log.d(TAG, "in onCreate()"); } @Override public void onStart() { super.onStart(); Log.d(TAG, "in onStart()"); } @Override public void onRestart() { super.onRestart(); Log.d(TAG, "in onReStart()"); } @Override public void onResume() { super.onResume(); Log.d(TAG, "in onResume()"); } @Override public void onPause() { super.onPause(); Log.d(TAG, "in onPause()"); } @Override public void onStop() { super.onStop(); Log.d(TAG, "in onStop()"); } @Override public void onDestroy() { super.onDestroy(); Log.d(TAG, "in onDestroy()"); } ...... ...... }
"LogCat" & DDMS
In the MainActivity
, we use static
method Log.d()
to write a "debug" message to the "LogCat" - this is the standard way of writing log messages in Android. System.out.println()
does not work under ADT.
LogCat is the Android Logging Utility that allows system and applications to output logging information at various logging levels. Each log entry consists of a time stamp, a logging level (V - verbose, D - debug, I - informational, W - warning , E - error), the process ID (pid
) from which the log came, a tag defined by the logging application itself, and the actual logging message.
You could use the various static methods in the java.Android.Log
class to log a message at various logging levels:
public static void v(String tag, String message); // Verbose public static void d(String tag, String message); // Debug public static void i(String tag, String message); // Informational public static void w(String tag, String message); // Warning public static void e(String tag, String message); // Error // where tag identifies the application
To show the LogCat panel, press "Alt+6" to show the "Android DDMS" panel. Logcat messages are displayed under "Devices | Logcat" tab.
From the LogCat panel, you can select a particular logging level, or filter based on pid, tag and log level.
Run the App
Run the application, and observe the message on the "LogCat" panel.
07-14 03:45:48.698: D/MainActivity:(752): in onCreate() 07-14 03:45:48.698: D/MainActivity:(752): in onStart() 07-14 03:45:48.708: D/MainActivity:(752): in onResume()
Click the "BACK" button:
07-14 03:46:29.559: D/MainActivity:(752): in onPause() 07-14 03:46:31.849: D/MainActivity:(752): in onStop() 07-14 03:46:31.899: D/MainActivity:(752): in onDestroy()
Run the application again from the "App Menu":
07-14 03:47:24.749: D/MainActivity:(752): in onCreate() 07-14 03:47:24.749: D/MainActivity:(752): in onStart() 07-14 03:47:24.759: D/MainActivity:(752): in onResume()
Click the "HOME" button to send the activity to the background, then run the app from the "App Menu" again. Notice that onDestroy()
is not called in this case.
07-14 03:48:06.369: D/MainActivity:(752): in onPause() 07-14 03:48:08.258: D/MainActivity:(752): in onStop() 07-14 03:49:14.650: D/MainActivity:(752): in onReStart() 07-14 03:49:14.650: D/MainActivity:(752): in onStart() 07-14 03:49:14.659: D/MainActivity:(752): in onResume()
Click the "PHONE" button, and then "BACK":
07-14 03:51:31.829: D/MainActivity:(752): in onPause() 07-14 03:51:36.700: D/MainActivity:(752): in onStop() 07-14 03:51:38.308: D/MainActivity:(752): in onReStart() 07-14 03:51:38.308: D/MainActivity:(752): in onStart() 07-14 03:51:38.329: D/MainActivity:(752): in onResume()
Take note that clicking the "BACK" button destroys the activity, but "HOME" and "PHONE" button does not.
Action Bar
[TODO]
Using Fragments
[TODO]
Example 7: Fragment
[TODO]
Handling Input Events
Like most of the programming languages (such as Java), Android adopts the event-handing model to process input events. (Read "Java Event Handling" for the basic concepts.)
An android.view.View
(UI component, widget or control) can register various event-listener objects via the setOnXxxListener()
. The event-listener object shall implement the appropriate OnXxxListener
interface and provide the various event handling methods.
public void setOnClickListener (View.OnClickListener l) public void setOnKeyListener (View.OnKeyListener l) public void setOnTouchListener (View.OnTouchListener l) public void setOnCreateContextMenuListener (View.OnCreateContextMenuListener l) public void setOnDragListener (View.OnDragListener l) public void setOnFocusChangeListener (View.OnFocusChangeListener l) public void setOnGenericMotionListener (View.OnGenericMotionListener l) public void setOnHoverListener (View.OnHoverListener l) public void setOnLongClickListener (View.OnLongClickListener l) public void setOnSystemUiVisibilityChangeListener (View.OnSystemUiVisibilityChangeListener l)
The OnXxxListener
s are defined as static nested class in android.view.View
.
[TODO] More